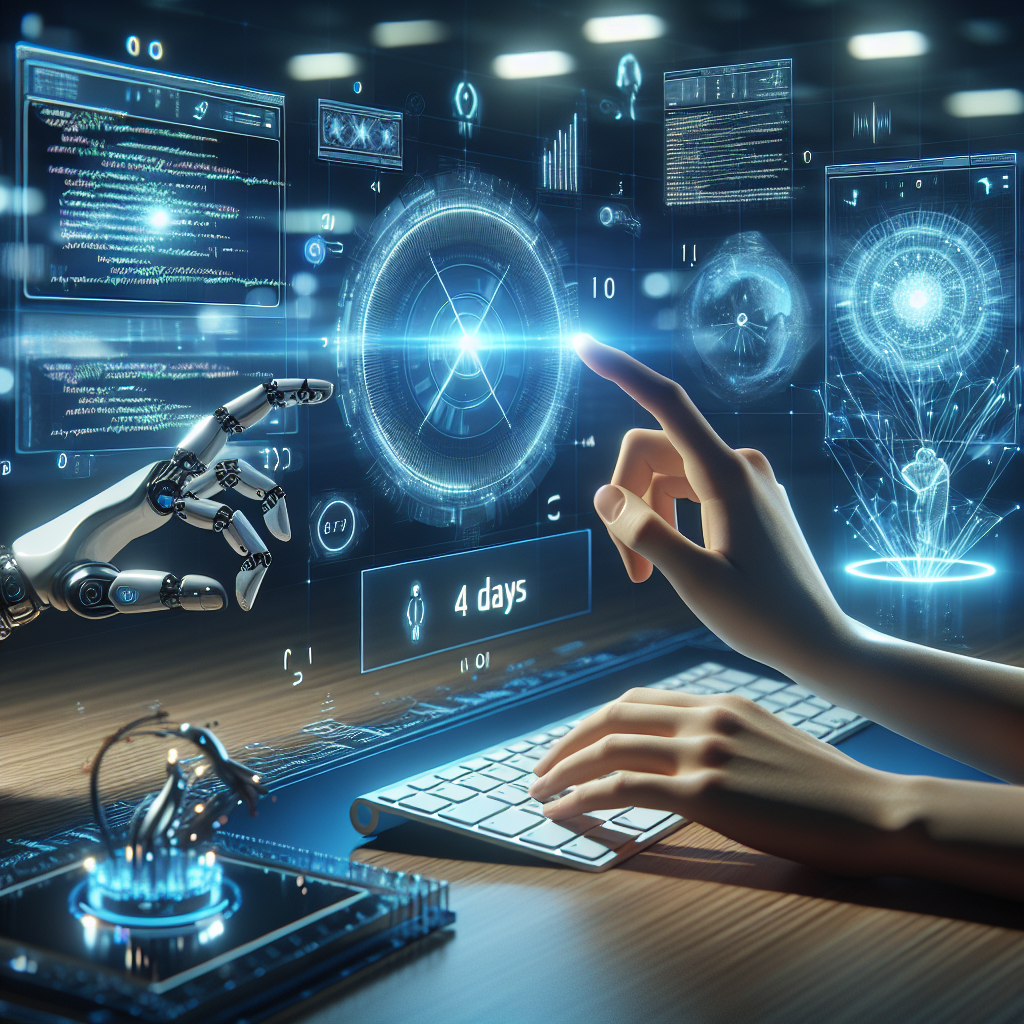
Lessons from a 14-Day AI-Powered Sprint
Imagine condensing months of coding into just two weeks. Our team embarked on an intensive 14-day sprint to find out, and the results were nothing short of eye-opening. From initial skepticism to newfound confidence, we discovered that AI isn’t just a coding shortcut—it’s a catalyst for reimagining the entire development process.
Executive Summary
In our 14-day experiment with AI-powered code generation, we witnessed a dramatic shift in productivity and code quality. Starting with a staggering 2,500 lines of code on day one, we refined our approach to produce 1,000 lines of high-quality, maintainable code by day 14. Key findings include the critical importance of human expertise in guiding AI, the necessity of rigorous testing, and the potential for AI to enhance domain-driven design practices. While challenges arose, particularly with .NET implementation, the sprint ultimately demonstrated AI’s capacity to significantly boost developer productivity when used judiciously.
The Journey: From Skepticism to Mastery
Day 1: Diving into the Deep End
As we kicked off our sprint, the air was thick with a mixture of excitement and trepidation. “I’m finally over the ‘I’m worried this won’t work’ phase,” one team member confessed, capturing the collective mood. We were about to push the boundaries of what we thought possible with AI-assisted coding.
Our chosen weapon? Cloude Sonnet 3.5, the latest large language model from Anthropic, integrated with Continue. Our team consisted of one seasoned developer,bringing a unique perspective to the table. We structured our sprint with daily stand-ups,programming sessions, and end-of-day retrospectives to keep us aligned and agile.
The first day was a whirlwind. We watched in awe as Claude churned out code at a dizzying pace. By day’s end, we had generated an impressive 2,500 lines of code. But as the initial euphoria subsided, a sobering question emerged: Was this code actually any good?
public class OrderProcessor{private readonly IOrderRepository _orderRepository;private readonly IPaymentService _paymentService;private readonly IInventoryService _inventoryService;private readonly IEmailService _emailService;
public OrderProcessor(IOrderRepository orderRepository, IPaymentService paymentService,
IInventoryService inventoryService, IEmailService emailService)
{
_orderRepository = orderRepository;
_paymentService = paymentService;
_inventoryService = inventoryService;
_emailService = emailService;
}
public async Task<bool> ProcessOrder(Order order)
{
// Check inventory
if (!await _inventoryService.CheckAvailability(order.Items))
{
return false;
}
// Process payment
var paymentResult = await _paymentService.ProcessPayment(order.TotalAmount, order.PaymentDetails);
if (!paymentResult.Success)
{
return false;
}
// Update inventory
await _inventoryService.UpdateInventory(order.Items);
// Save order
await _orderRepository.SaveOrder(order);
// Send confirmation email
await _emailService.SendOrderConfirmation(order);
return true;
}
}
This code snippet, while functional, lacked the nuanced architecture and error handling we’d typically implement. It was a wake-up call: AI could generate code rapidly, but it needed our expertise to make it production-ready.
The Learning Curve: Steeper Than Expected
As we delved deeper into the sprint, we quickly realized that effective AI-assisted coding demanded more than just prompting skills. We found ourselves on a crash course in advanced software engineering concepts:
- Mastering dependency injection patterns
- Exploring various persistence approaches, from traditional databases to event sourcing
- Diving deep into the intricacies of .NET and C#
This unexpected learning curve was both challenging and invigorating. It highlighted a crucial insight: AI is a powerful ally, but it’s not a substitute for solid programming fundamentals.
Key Insights and Lessons
1. Quality Over Quantity: The 1,000-Line Revelation
By day 14, our approach had undergone a dramatic transformation. “Day 14: 1000 lines of code,” announced the developer, pride evident in his voice. This wasn’t a step backward—it was a leap forward in our understanding of AI-assisted development.
We learned to guide the AI more effectively, focusing on generating smaller, more focused code segments that we could easily review and integrate. This approach led to cleaner, more maintainable code that adhered to our architectural vision.
2. Testing: The Unsung Hero of AI-Assisted Development
As our codebase grew, so did our appreciation for rigorous testing. We implemented a comprehensive testing strategy, including unit tests, integration tests, and behavior-driven development (BDD) scenarios.
gherkin
Feature: Order Processing
Scenario: Successfully process a valid order
Given a customer has items in their cart
And the items are in stock
And the customer's payment information is valid
When the customer places the order
Then the order should be successfully processed
And the inventory should be updated
And a confirmation email should be sent to the customer
These tests became our safety net, catching subtle bugs and ensuring that our AI-generated code met all functional requirements and edge cases.
3. Domain-Driven Design: AI’s Unexpected Strength
One of the most surprising outcomes was how well AI adapted to domain-driven design principles. With careful prompting, we were able to generate code that beautifully encapsulated complex business logic within bounded contexts.
public class OrderAggregate : AggregateRoot
{
private List _items = new List();
public IReadOnlyCollection Items => _items.AsReadOnly();
public OrderStatus Status { get; private set; }
private OrderAggregate() { } // For ORM
public static OrderAggregate Create(Guid customerId)
{
var order = new OrderAggregate
{
Id = Guid.NewGuid(),
CustomerId = customerId,
Status = OrderStatus.Created
};
order.AddDomainEvent(new OrderCreatedEvent(order.Id, customerId));
return order;
}
public void AddItem(ProductId productId, int quantity, Money unitPrice)
{
if (Status != OrderStatus.Created)
throw new OrderException("Cannot add items to a non-draft order.");
var existingItem = _items.FirstOrDefault(i => i.ProductId == productId);
if (existingItem != null)
{
existingItem.IncreaseQuantity(quantity);
}
else
{
_items.Add(new OrderItem(productId, quantity, unitPrice));
}
AddDomainEvent(new OrderItemAddedEvent(Id, productId, quantity, unitPrice));
}
// Other methods like RemoveItem, PlaceOrder, etc.
}
This code demonstrates how AI can generate complex domain models that encapsulate business rules and maintain invariants, a cornerstone of DDD.
4. Productivity Gains: Beyond Google-Fu
As we honed our AI collaboration skills, we noticed a significant uptick in productivity. “My productivity at this point is over what I could do with Google for sure.”. AI wasn’t just faster at generating code; it was helping us explore solution spaces we might not have considered otherwise.
5. Persistence Pays Off: Overcoming Late-Stage Hurdles
Our journey wasn’t without its challenges. In the final days of the sprint, we hit a roadblock with some complex .NET implementations. It took an additional three days of intense work to ensure our testing was rock-solid. This experience underscored a vital lesson: AI-assisted development doesn’t eliminate the need for problem-solving skills and perseverance.
Limitations and Challenges
While our sprint was largely successful, we encountered several limitations of AI-assisted coding:
- Context Understanding: AI sometimes struggled with broader project context, requiring careful prompt engineering.
- Inconsistent Naming Conventions: We needed to standardize naming across AI-generated code manually.
- Over-Engineering: The AI occasionally produced overly complex solutions for simple problems.
- Security Concerns: We had to be vigilant about potential security vulnerabilities in generated code.
Actionable Takeaways for Implementing AI-Assisted Development
- Start Small: Begin with contained features or modules to get a feel for AI collaboration.
- Invest in Prompt Engineering: Develop a library of effective prompts tailored to your project’s needs.
- Emphasize Code Review: Implement rigorous peer review processes for AI-generated code.
- Integrate Continuous Testing: Set up automated testing pipelines to catch issues early.
- Balance AI and Human Creativity: Use AI for boilerplate and repetitive tasks, but rely on human insight for architecture and complex logic.
The Future of AI in Software Development
As we wrapped up our sprint, discussions inevitably turned to the future. The rapid advancements in GPU technology—with next-gen cards boasting up to 32GB VRAM and datacenter solutions pushing 1000W with 288GB VRAM—hint at the growing computational demands of AI-assisted development.
These hardware trends suggest that “VRAM per dollar” will become a crucial metric for development teams looking to leverage AI effectively. However, this also raises important questions about the environmental impact and sustainability of AI-powered development practices.
Conclusion: A New Era of Collaborative Coding
Our 14-day sprint into AI-assisted development was a rollercoaster of challenges and breakthroughs. We emerged with not just a functional codebase, but a transformed perspective on the role of AI in software engineering.
As one team member eloquently put it, “I’m proud of the output even if the path to get there was really squiggly.” This sentiment captures the essence of our journey—a winding path that led to unexpected innovations and a deeper appreciation for the symbiosis between human creativity and artificial intelligence.
The future of software development is neither purely human-driven nor entirely automated. It’s a collaborative dance between developers and AI, each amplifying the other’s strengths. As we continue to refine this partnership, we stand on the brink of a new era in coding—one where the limits of what’s possible are constantly expanding, and where the journey of creation is as rewarding as the destination.